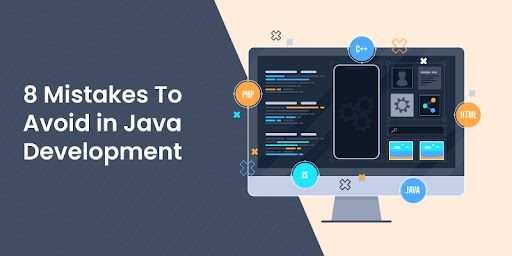
Java, like that perfectly brewed cup of joe, can keep you running all night when you have a pressing deadline. But just like forgetting the creamer can turn your morning pick-me-up into a bitter disappointment, there are a few pitfalls that can leave your Java development feeling, well, a little burnt.
I've been in the trenches of Java for years, and let me tell you, I've seen it all: concurrency chaos, memory leaks that would rival the biggest spendthrift, and exception handling that could make your head spin faster than a ballerina on Red Bull.
Well, this is what I’m here to talk about. To share some war stories - and, more importantly, the lessons I learned from them - to help you avoid these common Java mistakes.
Think of this as your personal "Java JEDI training manual," packed with tips and tricks to make your code sing like a rockstar (minus the bugs). So, grab your keyboard, fire up your IDE, and get ready to write some clean, efficient, and maintainable Java code.
Mistake #1: Null Pointer Problems
Let's talk about the bane of many Java developers' existence: the NullPointerException.
This infamous little critter pops up whenever I try to access a method or property on an object that, well, doesn't exist. In Java-speak, it means you're referencing a null value.
Here's a common scenario:
In this example, the greetUser method attempts to call toUpperCase() on the name variable. But what happens if we call greetUser before setting a value for name? Since name is initialized to null by default, we're trying to call a method on a non-existent object, causing the NullPointerException.
Here’s the fix:
- Guard Clauses: These are conditional statements placed at the beginning of your methods to check if a variable might be null. If it is, handle the situation gracefully (e.g., return a default value, throw a custom exception).
- Optional Objects: Java 8 introduced the Optional class, a powerful tool for dealing with potentially null values. You can use methods like isPresent() to check if a value exists before accessing it.
This significantly reduces the risk of NullPointerExceptions and writes more robust Java code. A little extra caution at the beginning can save you a lot of debugging headaches later.
Mistake #2: Concurrency Issues
Concurrency is the shiny object that promises faster execution times, but it can also morph into a monstrous spaghetti junction of tangled threads if not handled carefully.
When I was optimizing a web application a couple of years back, the code looked simple - a single thread processing user requests.
But under load, performance plummeted. Digging deeper, I discovered the culprit: a database call within the request processing logic. Each request had to wait for the previous one to finish its database interaction, creating a bottleneck.
The answer? Concurrency. I rewrote the application to use multiple threads, each handling a separate user request. It worked. Kind of. Suddenly, we were plagued by strange data inconsistencies.
Transactions weren't being isolated properly, leading to race conditions where multiple threads tried to modify the same data at the same time.
Lessons learned:
Synchronized Blocks: These are essential for ensuring thread-safe access to shared resources. Imagine a synchronized block as a metaphorical "mutex" – only one thread can enter at a time, guaranteeing data integrity.
Thread-Safe Collections: The Java Collections Framework offers thread-safe alternatives to standard collections like ArrayList. Using classes like ConcurrentHashMap ensures consistent behavior when accessed by multiple threads.
Remember, with great power comes great responsibility (and a whole lot of synchronized blocks).
Mistake #3: Keeping Your Heap in Check
Memory leaks are the silent assassins of Java applications. They creep in slowly, gobbling up precious memory resources until your once-sprightly program resembles a sluggish sloth on a sugar crash.
I was working on a complex data processing application that involved loading large datasets into memory for manipulation. Everything seemed fine during initial testing, but after running for a few hours, the application would grind to a halt with an "OutOfMemoryError." Yikes.
The culprit? In my haste, I had neglected to properly close database connections and release resources after processing each dataset. These unclosed connections lingered in memory, slowly draining the heap's capacity. Here’s what I did:
- Resource Management: Java's try-with-resources statement is your best friend here. It ensures that resources are automatically closed, even if exceptions occur.
- Profiling Tools: Use tools like JVisualVM to identify objects that are not being garbage collected. This can help pinpoint the source of memory leaks and take corrective action.
Mistake #4: Unnecessary Exceptions
I swear debugging can sometimes feel like playing detective. Sifting through clues and evidence until you finally catch your culprit—or, in our case, fix that darn bug.
While exceptions are a necessary evil for robust error handling, encountering a cascading wall of uninformative exception messages can leave you feeling like you're deciphering ancient hieroglyphics.
An error occurs deep within the logic, but the exception bubbles up through multiple methods, each adding its own stack trace layer. By the time it reaches the top level, the original error message is buried under a mountain of technical jargon—not exactly helpful when I start debugging. Here’s my secret:
Specific Exception Handling: Instead of catching broad exceptions like Exception, use more specific types like IOException or NullPointerException. This provides clearer indications of the root cause.
Custom Exceptions: For errors specific to your application logic, create custom exception classes that encapsulate meaningful error messages.
Mistake #5: Overengineering
As a developer, I often get caught up in the allure of over-engineering. Complex design patterns and intricate architectures can be incredibly tempting. However, I’ve learned through experience that the simplest solution is often the best. It’s not about being lazy; it’s about prioritizing clarity and maintainability over unnecessary complexity.
Fresh out of Java boot camp, my head was buzzing with knowledge about Singleton patterns and dependency injections. My first project was a user management system—seemingly straightforward. But, I went overboard with a layered architecture filled with abstract classes and interfaces.
On paper, it looked impressive. In practice, it was a mess. Adding a simple feature turned into a week-long ordeal. It was a lesson learned the hard way: simpler is often better.
Focus on Readability: Write code that is easy to understand. Future-me (or anyone else who inherits my code) will appreciate it. Clear variable names, proper indentation, and useful comments are essential.
Start Simple, Refactor Later: Avoid the trap of premature optimization. Begin with a straightforward implementation and refactor as the project evolves. Keep in mind the principle of YAGNI ("You Ain't Gonna Need It"). Don’t overbuild for features that might never be needed.
By keeping my code clear and simple, I make my Java creations more robust and easier to maintain. It made me a better developer, and I hope it helps you too.
Mistake #6: Logging Mismanagement
Logs are crucial for understanding the inner workings of our applications, but without proper management, they can become a chaotic mess. Here’s how I make my logs more effective and useful for debugging.
Log Levels: Use different log levels (DEBUG, INFO, WARN, ERROR) to categorize the severity and importance of log messages. This helps filter out unnecessary information and focus on what’s critical.
Meaningful Messages: Avoid generic log messages. Include details like method names, variable values, and specific context to make it easier to identify issues.
Mistake #7: External Dependency
Java development thrives on a vast ecosystem of libraries and frameworks. These external dependencies can be powerful tools, but managing them effectively is crucial. Let's talk about some pitfalls to avoid and strategies to keep your project's dependencies under control.
In a previous project, we heavily relied on a third-party library that hadn't been updated in years. This outdated dependency introduced compatibility issues and security vulnerabilities. Updating the library caused cascading issues throughout the codebase, leading to a week of frantic troubleshooting. Here’s what I’ve learned:
Version Management: Use dependency management tools like Maven or Gradle to manage the versions of your dependencies. This ensures consistency and avoids conflicts.
Stay Up-to-Date: While stability is important, don't neglect security updates and bug fixes for your dependencies. Schedule regular reviews and updates to keep your project in tip-top shape.
Evaluate Alternatives: The Java ecosystem is constantly evolving. Don't be afraid to explore newer, better-maintained libraries if they offer a better fit for your needs.
Mistake #8: Overlooking Documentation
The often-dreaded but undeniably valuable companion to any project. While lines of code might be the building blocks, clear and concise documentation acts as the instruction manual and roadmap for anyone interacting with your code.
Documentation do's and don'ts:
Document Code Structure: Provide an overview of the project architecture, including modules, packages, and major components. This helps developers navigate through the codebase and locate specific functionalities.
Document Functionality: Document each function, method, or class to explain its purpose, inputs, outputs, and expected behavior. Use clear and concise language to ensure comprehension.
Include Examples: Use code snippets, usage examples, and scenarios to illustrate how to use different parts of your codebase effectively.
Update Regularly: Keep documentation up to date with the latest code changes. Version control your documentation alongside your codebase to ensure accuracy and relevance.
Use Consistent Formatting: Maintain a consistent style and format throughout your documentation to enhance readability and usability.
Clear documentation benefits not only future developers but also your present self as you revisit your code months or years later.
Wrapping Up!
I am constantly learning and growing as a dev. Every project throws new challenges my way, and each mistake (believe me, I've made a few!) becomes a valuable lesson. By sharing these insights with you, I hope to help you avoid some of the common pitfalls I've encountered and empower you to write exceptional Java code.